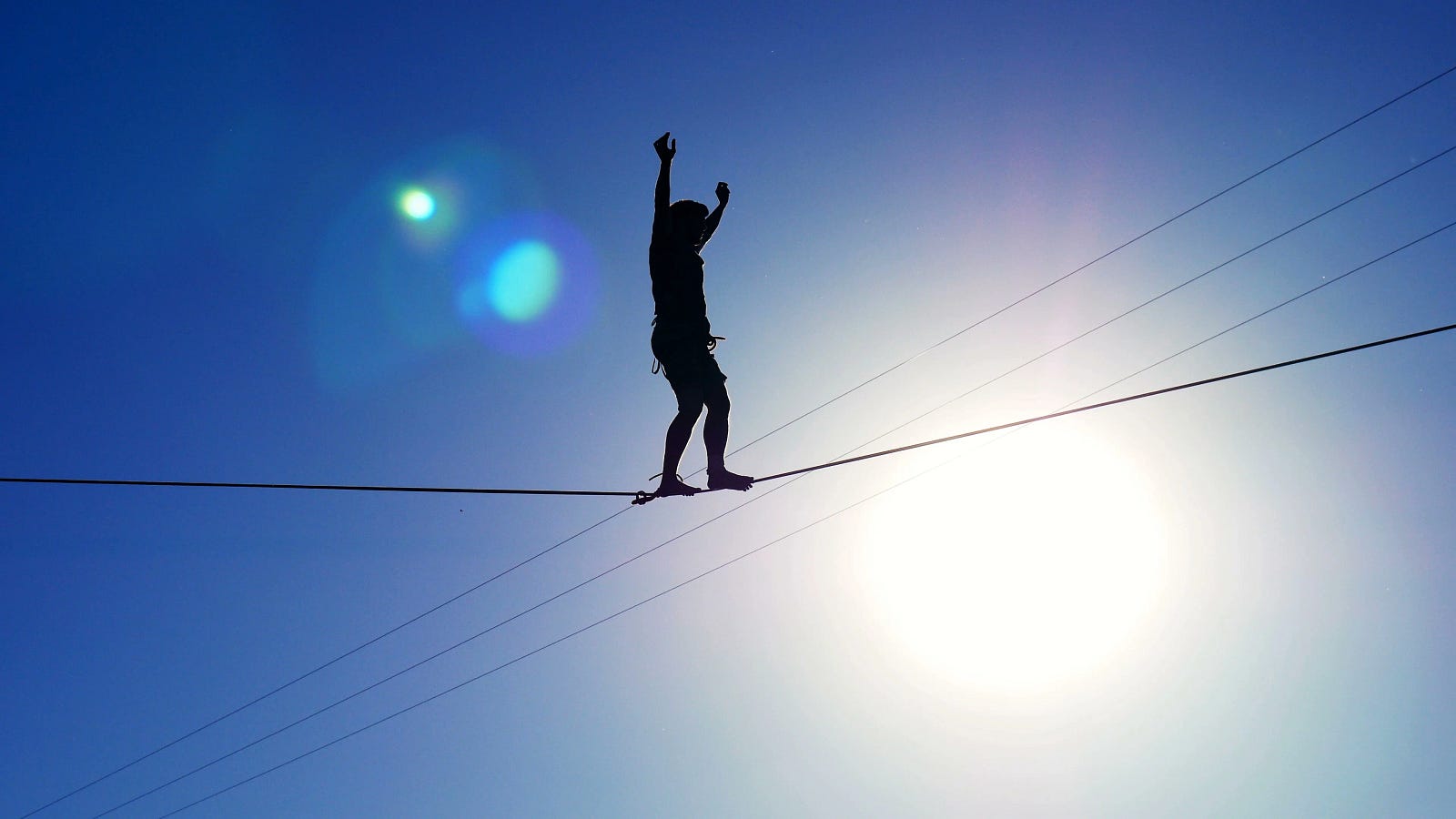
Ah yes, Phil Karlton’s two hard things in computer science: cache invalidation and naming things. They have puzzled and perturbed developers for years. But may I suggest a third — describing your problem to Google or Chat GPT?
Imagine trying to describe a UI bug. You’re standing in front of a M.C. Escher looking website, telling your friend on the other end of the phone who cannot see it, that it looks like a menu at the top of the page, but not quite, it’s opening in unexpected ways and leading to surprising locations that should not even exist. You’re not alone if this scenario makes you want to throw away your computer.
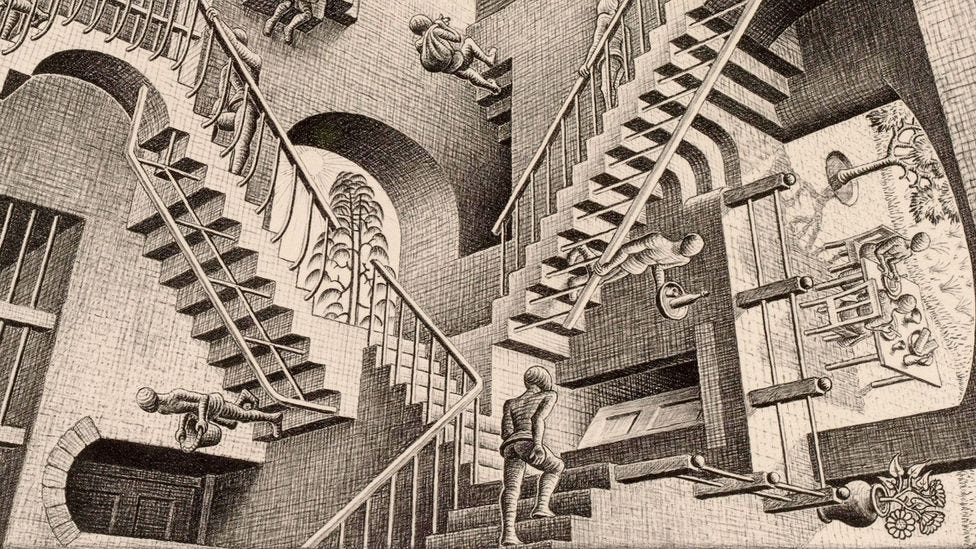
Or, consider a server that’s giving you the silent treatment. It’s like trying to explain why your cat won’t eat its favorite food anymore. Is it bored? Is it mad at you? Is it planning a world conquest?
In both scenarios, you’re dealing with an ambiguous issue that can be tricky to pin down. In the case of a UI bug, what you need is to accurately describe what’s wrong. What’s supposed to happen, what’s actually happening, and how do they differ? This difference is actually what you are looking for! A phrase like “HTML form input not triggering JavaScript event” might yield better results than “website not working.”
For a non responsive server, check the error messages, if any, and observe the circumstances. Look for patterns. Then, translate these observations into a query like “Apache server timeout with heavy load” or “Node.js server not responding after database query.”
Problem solving in software development isn’t just about fixing things. It’s also about asking the right questions and learning how to communicate effectively with our tools. Just like with naming things and cache invalidation, it can be tough, but that’s what makes it one of the great challenges of our craft. And who knows? You might just end up having a heart-to-heart with your favorite search engine or AI model.
The Shakespearean Dilemma in Coding: What’s in a Name?
Phil Karlton hit the nail on the head when he declared naming things as one of the two hardest problems in computer science. It’s a delicate balancing act — trying to give a variable a name that’s sufficiently descriptive but not as long as a Victorian era novel.
Take, for instance, a Boolean variable that signifies whether a user is above 18 or not. You could call it “isEighteen”, but that’s ambiguous — does it mean they’re exactly 18 or over 18? You might go for “userIsOverEighteen”, which is crystal clear but also quite lengthy. “ageValid” is shorter, but it could refer to other age validations as well. Each option has its merits and demerits, and picking the best one often involves a lot of chin scratching and caffeine.
Picking the right name is vital because well-named variables can make your code dramatically easier to understand and maintain. A good name can be a beacon of clarity in a sea of syntactical complexity.
A great tip is using naming conventions or patterns to give you extra information about variables. For example, prefixing DOM related variables with a dollar sign, like $inputFields = document.querySelectorAll(“input”), immediately tells you that the variable relates to an element or elements from the DOM. Such conventions can be a tremendous help when you’re navigating through hundreds of lines of code, searching for that one mischievous variable wreaking havoc in your beautiful codebase.
Function Naming Conundrums and the Tale of Utils.js
The difficulty of naming extends its treacherous tentacles even to functions or methods. If you find it challenging to name a function, it could be a sign that the function is doing too much. It’s like those kitchen appliances that promise to do everything: chop, grate, cook, clean, and order the groceries needed for your next meal. While it sounds impressive, you realize that it would be lucky if it could slice a ripe banana.
For instance, consider a JavaScript function like this:
function calculateAndPrintInvoice(user, items) {
// calculates total, applies discounts, prints invoice…
}
If you’re scratching your head over whether to name it calculateInvoice, printInvoice, or calculateAndPrintInvoice, it’s likely because the function is multitasking like an overworked intern. A better approach might be to split it into smaller, single responsibility functions like calculateInvoice and printInvoice. This not only simplifies naming but also makes your code more modular and manageable.
The same conundrum extends to naming files in your project. Ever come across a file named utils.js? It’s like the junk drawer of your codebase — a mysterious place where you put everything you’re not quite sure where to categorize. Or how about the infamous common folder? It’s like the Bermuda Triangle of your project, where random bits of code disappear, never to be seen again.
When you’re having a hard time naming something, maybe it’s not the naming that’s hard, but figuring out what your function or file is really doing or containing. Once you’re clear on that, the perfect name might just pop up.
Deciphering Code Hieroglyphics: Why Explaining Bugs Is a Bugger
With a grasp of the trials of naming, let’s venture into another perplexing domain: explaining your code problems or bugs. It’s a bit like trying to describe a dream — it all made sense in your head, but when you try to put it into words, it comes out like a bizarre mix of sci-fi and abstract art.
For starters, code is inherently abstract. It’s a sequence of symbols that performs tasks, manipulates data, and interacts with other code in often complex ways. Explaining a problem requires you to transform this abstract, symbolic representation into clear, concrete language. It’s like trying to describe color to your best friend who can only see in black and white.
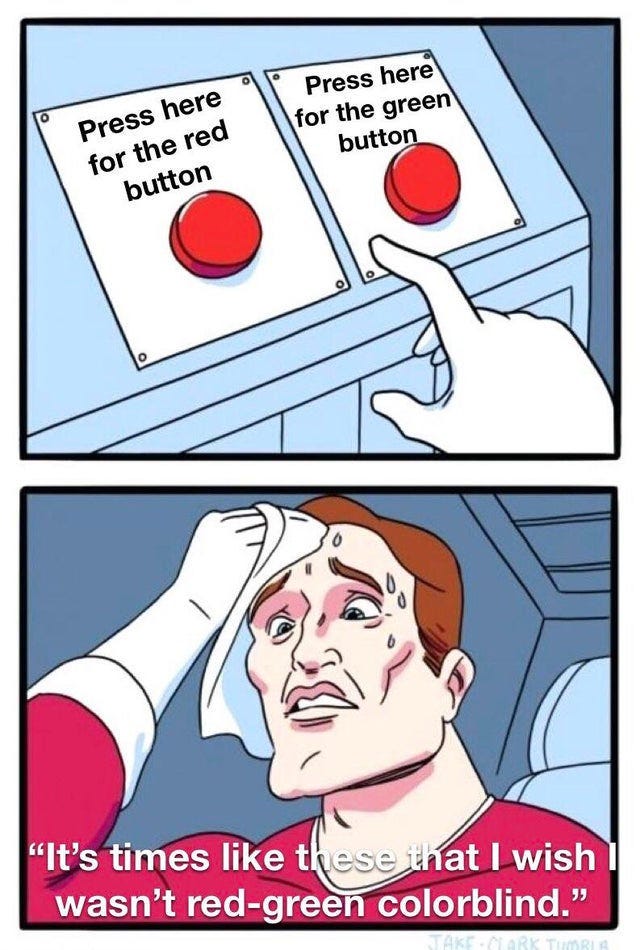
What makes it even trickier is that bugs often involve many interconnected parts of your code. It’s like a mystery novel, where minor characters and seemingly irrelevant details end up being crucial to the plot. The problem isn’t just that the function returns an error; it’s how the data got to the function, what state the system was in, what the user did three steps before, and why your coffee cup was empty at the critical moment.
And then there’s the infamous heisenbug — a bug that changes or disappears when you try to study it. These digital gremlins make explaining the problem feel like trying to nail jelly to a wall.
Thus, describing a bug requires more than merely pointing out its flaws. Telling the tale of your code involves using variables and functions as the characters, an algorithm or process as the storyline, unexpected behavior as the turns, and the bug as the conclusion. And it typically requires a few revisions to get it right, just as with any good story. A tale is ready to be told about every defect. Make sure yours is a hit.
Code Whispers: Describing Bugs Like a Detective
You’re going to need to harness your inner Sherlock Holmes when it comes to describing a bug in order to identify the solution. assemble the facts, state the facts, and briefly and precisely state your concern. But how, I hear you ask? Let’s look at two real world instances, one from the frontend development community and the other from the shadowy backend regions.
Frontend Example:
Imagine you’re building a website using React, and for some ungodly reason, a button isn’t triggering its onClick event. A poor way to Google this would be “button not reacting.” It’s too vague and could apply to myriad issues. Instead, provide specific details about the issue and any error messages. A better search might be “React onClick event not triggering on button with custom component.” This clearly states the technology, the event type, the element in question, and a hint at the potential cause.
Backend Example:
Now, consider you’re working with a Node.js server, and you keep getting an ECONNREFUSED error when you try to connect to your MySQL database. Don’t just search for “database connection error”; that’s like asking for directions in a city without providing a specific address. A more refined and detailed search would be “ECONNREFUSED error when connecting to MySQL database in Node.js.” This spells out the specific error, the technology stack involved, and the operation that’s failing.
Final Thoughts: From Novice to Sage
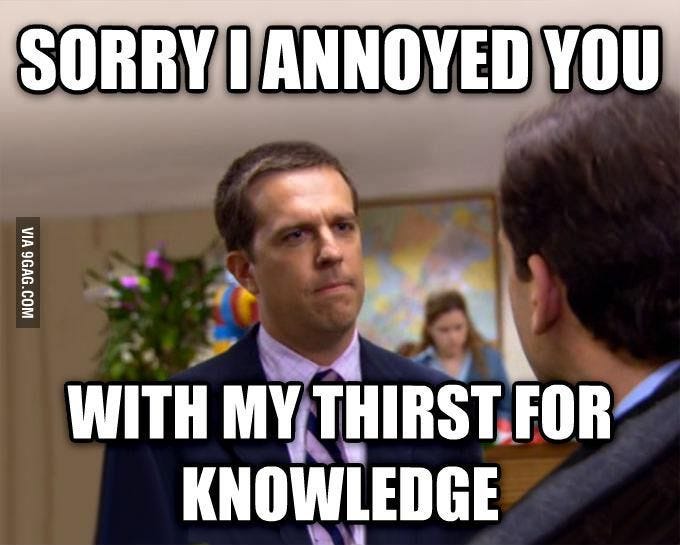
Let me share a slice of my experience as a rookie developer. Early on, I recognized that whenever I had a problem, if I took the time to do some research before turning to my senior colleagues with questions, two important things happened.
Firstly, they noticed my dedication and ability to understand the problem. I wasn’t just throwing out questions like “Why is my screen black?” (without turning my computer on) at them. Instead, I was presenting well researched queries and demonstrating my potential to grow and learn independently.
Secondly, the preliminary research often helped me eliminate many potential culprits for my issue. More often than not, it acted like a sieve, filtering out the red herrings and leaving me with a handful of potential leads that would help solve the problem within minutes.
So whether you’re navigating the wild seas of Google, diving into the depths of Stack Overflow, or conversing with a language model like ChatGPT, the art of asking questions and searching for answers is a skill honed with practice, patience, and a sprinkle of wit. Your search may sometimes lead to a comedic episode of ‘Finding Nothing,’ but even that is a clue to guide you on this exciting, sometimes frustrating, but always rewarding journey of software development.